Description
A "While..Do" loop is used to perform a group of instructions while a
certain condition remains true. The syntax of a "while..do" loop is:
while <expression> do
<statement>;
The expression is evaluated and if found to be true the statement is
executed. This continues until the expression is found to be false. For
example:
While not MovementFile.EndOfFile do
begin
MovementFile.Readln(XCoord,YCoord);
XYAxis.MoveTo(XCoord,YCoord);
end;
This checks to insure there is information remaining in the
MovementFile (a TFile accesses files on the PC). If information is still in the file that information is retrieved
and the motion system moves to that location until the information is all
used. This will iterate various numbers of times depending on how
many coordinate pairs are in the file. Note also that if there is no
information in the file this loop never executes at all, i.e. it handles
the empty case.
While loops are best for iteration where the number of times desired
may be unknown and the number of times might be none at all.
There are some cases where an infinite loop is required. This is is most simply done in with
a while..do loop with a conditon being the boolean constant "true"
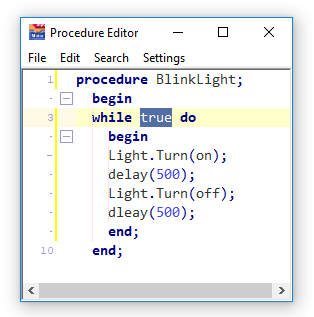
True is always true;
There are some synchronizing tasks where the objective is to do
nothing at all until a certain event occurs and then to proceed with
an operation. For example you might want to turn on a glue gun after
the x axis has passed 5:
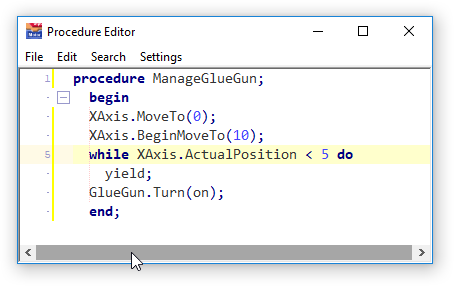
Note the "yield" instruction inside the otherwise "empty" while
statement. For some single-tasking systems, doing nothing is an
option but in a multitasking system doing nothing is quite selfish.
The controller uses a cooperative multitasking architecture. If a
task is simply waiting it is important to yield to other system activities.
Yield is a multitasking management procedure that directs a task
to give up execution because the application developer knows that
nothing interesting will happen until the next controller sample. Execution will
return to this procedure in the next millisecond if the controller sample rate
is set to 1 kHz. It is extremely important that the yield is present.
A task must not
attempt to control the CPU for longer than it's share of the sample. Forgetting the yield instruction in
an indefinite loop can cause the controller to lock. Principles of multitasking will be discussed later at greater
length however the point to be made here is that
there are usually no empty loops.